|
 |
floating point source code |
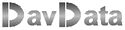 |
 |
back to article
unit Unit1;
interface
uses
Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms,
StdCtrls, Buttons, ExtCtrls;
type
TForm1 = class(TForm)
number: TEdit;
bin: TStaticText;
Label1: TLabel;
singleBtn: TSpeedButton;
doubleBtn: TSpeedButton;
Label3: TLabel;
GOBtn: TButton;
clearBtn: TSpeedButton;
Label2: TLabel;
exponent: TStaticText;
sign: TStaticText;
StaticText1: TStaticText;
coef: TStaticText;
Label4: TLabel;
Label5: TLabel;
Label6: TLabel;
Label7: TLabel;
Label8: TLabel;
Label9: TLabel;
procedure FormCreate(Sender: TObject);
procedure FormKeyPress(Sender: TObject; var Key: Char);
procedure GOBtnClick(Sender: TObject);
procedure clearBtnClick(Sender: TObject);
procedure singleBtnClick(Sender: TObject);
private
{ Private declarations }
public
{ Public declarations }
end;
var
Form1: TForm1;
implementation
{$R *.dfm}
type TA = array[0..7] of byte;
PA = ^TA;
const kbfilter = ['0'..'9',#8,'.','-'];
msg71 = 'E=[E7..E0]';
msg72 = 'E=[E10..E0]';
msg81 = 'M=[M1..M23]';
msg82 = 'M=[M1..M52]';
msg91 = 'value = (-1)^S*(1.M)*2^(E-127)';
msg92 = 'value = (-1)^S*(1.M)*2^(E-1023)';
procedure TForm1.FormCreate(Sender: TObject);
begin
decimalseparator := '.';
label7.Caption := msg71;
label8.Caption:= msg81;
label9.Caption := msg91;
end;
procedure cleartext;
begin
with form1 do
begin
bin.caption := '';
sign.Caption := '';
exponent.Caption := '';
coef.caption := '';
end;
end;
procedure TForm1.FormKeyPress(Sender: TObject; var Key: Char);
begin
if activecontrol = number then
begin
if key in kbfilter then
begin
if key = '.' then
if pos(key,number.text) > 0 then key := #0;
end
else key := #0;
end;
end;
procedure TForm1.GOBtnClick(Sender: TObject);
//show format
var p : PA;
i,j : byte;
s,t : string;
f32 : single;
f64 : double;
begin
s := '';
cleartext;
try
if singleBtn.Down then
begin
f32 := strtofloat(form1.number.text);
p := PA(@f32);
for j := 3 downto 0 do
for i := 7 downto 0 do s := s + chr(((p^[j] shr i) and 1) + ord('0'));
sign.Caption := s[1];
t := '';
for i := 2 to 9 do t := t + s[i];
exponent.Caption := t;
t := '';
for i := 10 to 32 do t := t + s[i];
coef.Caption := t;
end
else
begin
f64 := strtofloat(number.Text);
p := PA(@f64);
for j := 7 downto 0 do
for i := 7 downto 0 do s := s + chr(((p^[j] shr i) and 1) + ord('0'));
sign.Caption := s[1];
t := '';
for i := 2 to 12 do t := t + s[i];
exponent.Caption := t;
t := '';
for i := 13 to 64 do t := t + s[i];
coef.Caption := t;
end;
bin.Caption := s;
except
bin.caption := 'format error';
end;
end;
procedure TForm1.clearBtnClick(Sender: TObject);
begin
cleartext;
number.text := '';
end;
procedure TForm1.singleBtnClick(Sender: TObject);
begin
cleartext;
if singleBtn.Down then
begin
label7.Caption := msg71;
label8.Caption:= msg81;
label9.Caption := msg91;
end
else
begin
label7.Caption := msg72;
label8.Caption:= msg82;
label9.Caption := msg92;
end;
end;
end.
|
|